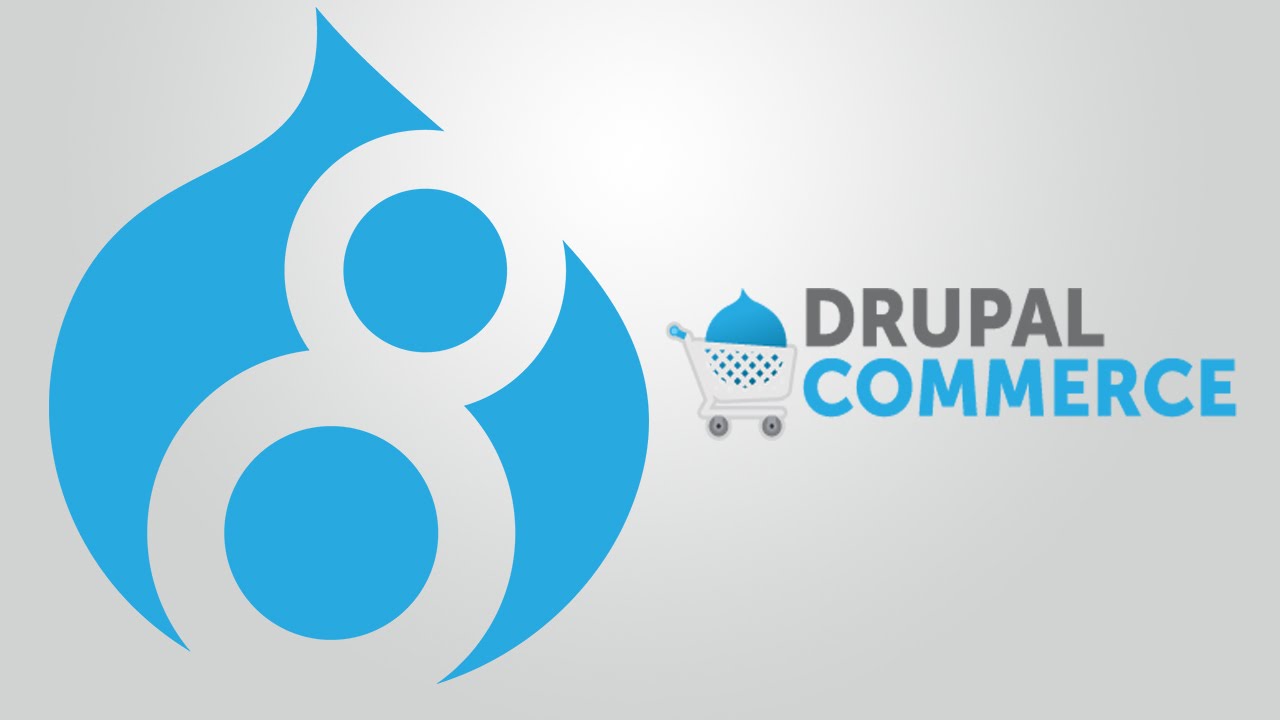
Bu makalede, Drupal 8'de Drupal Commerce için nasıl bir özel ödeme bölmesi oluşturabileceğimizi göstereceğim. Bu amaçla yapılandırma formuna sahip bir ödeme bölmesi ve kullanıcıların siparişlerine kupon ekleme olanağı yaratacağız.
Drupal commerce özel ödeme bölmesi oluşturma
namespace Drupal\module_name\Plugin\Commerce\CheckoutPane; use Drupal\commerce_checkout\Plugin\Commerce\CheckoutFlow\CheckoutFlowInterface; use Drupal\commerce_checkout\Plugin\Commerce\CheckoutPane\CheckoutPaneBase; use Drupal\commerce_checkout\Plugin\Commerce\CheckoutPane\CheckoutPaneInterface; use Drupal\Core\Entity\EntityTypeManagerInterface; use Drupal\Core\Form\FormStateInterface; /** * Provides the coupons pane. * * @CommerceCheckoutPane( * id = "coupons", * label = @Translation("Redeem Coupon"), * default_step = "order_information", * ) */ class CommerceCoupons extends CheckoutPaneBase implements CheckoutPaneInterface { /** * {@inheritdoc} */ public function __construct(array $configuration, $plugin_id, $plugin_definition, CheckoutFlowInterface $checkout_flow, EntityTypeManagerInterface $entity_type_manager) { parent::__construct($configuration, $plugin_id, $plugin_definition, $checkout_flow, $entity_type_manager);
Bu, özel ödeme bölmesinin bir 'kimlik kuponu' sahibi olacağı ve Ödül kuponu olarak etiketleneceği ve varsayılan olarak sipariş bilgi adımında belirleneceği anlamına gelir. Önce bölmemizin ayarlarını (yapılandırma) formunu oluşturuyoruz. Bu amaçla, kullanıcının sipariş üzerine yalnızca bir kupon kullanabileceği şekilde ayarlamamızı yapacağız. Bunun için, dört fonksiyon uygulamalıyız. Önce, varsayılan yapılandırmamızı kuracağız.
/** * {@inheritdoc} */ public function defaultConfiguration() { return [ 'single_coupon' => FALSE, ] + parent::defaultConfiguration(); }
Ardından yapılandırma formumuzun özetini uygulayacağız.
/** * {@inheritdoc} */ public function buildConfigurationSummary() { $summary = !empty($this->configuration['single_coupon']) ? $this->t('Single Coupon Usage on Order: Yes') : $this->t('Single Coupon Usage on Order: No'); return $summary;
Sonra yapılandırma formumuzu oluşturacağız.
/** * {@inheritdoc} */ public function buildConfigurationForm(array $form, FormStateInterface $form_state) { $form = parent::buildConfigurationForm($form, $form_state); $form['single_coupon'] = [ '#type' => 'checkbox', '#title' => $this->t('Single Coupon Usage on Order?'), '#description' => $this->t('User can enter only one coupon on order.'), '#default_value' => $this->configuration['single_coupon'], ]; return $form; }
Sonrasında bu ayaraları kayıt edin.
/** * {@inheritdoc} */ public function submitConfigurationForm(array &$form, FormStateInterface $form_state) { parent::submitConfigurationForm($form, $form_state); if (!$form_state->getErrors()) { $values = $form_state->getValue($form['#parents']); $this->configuration['single_coupon'] = !empty($values['single_coupon']); } }
Ödeme akışının düzenleme sayfasında nasıl görüneceğini görebilirsiniz. Sonra, bölme formumuzu oluşturacağız. Yapılandırmamızı kontrol edeceğiz ve siparişte bir kupon uygulanmışsa ve tek kupon için yapılandırma ayarına sahipse form göstermeyeceğiz.
/** * {@inheritdoc} */ public function buildPaneForm(array $pane_form, FormStateInterface $form_state, array &$complete_form) { $order_has_coupons = $this->order->coupons->referencedEntities(); if($this->configuration['single_coupon'] && $order_has_coupons){ return $pane_form; } $pane_form['coupon'] = [ '#type' => 'textfield', '#title' => $this->t('Coupon'), '#default_value' => '', '#required' => FALSE, ]; return $pane_form; }
Bundan sonra, kuponlarımızın geçerliliğini uygulayacağız ve geçerli bir kuponun sağlanıp sağlanmadığını ve bu siparişe uygulanabilir olup olmadığını kontrol edeceğiz.
/** * {@inheritdoc} */ public function validatePaneForm(array &$pane_form, FormStateInterface $form_state, array &$complete_form) { $values = $form_state->getValue($pane_form['#parents']); if(!empty($values['coupon'])){ $coupon_code = $values['coupon']; /* @var \Drupal\commerce_promotion\Entity\Coupon $commerce_coupon */ $commerce_coupon = $this->entityTypeManager->getStorage('commerce_promotion_coupon')->loadByCode($coupon_code); $valid = false; if($commerce_coupon){ $promotion = $commerce_coupon->getPromotion(); $valid = $promotion->applies($this->order) ? true : false; if($valid){ foreach($this->order->coupons->referencedEntities() as $coupon){ if($commerce_coupon->id() == $coupon->id()){ $form_state->setError($pane_form, $this->t('Coupon already applied to order.')); } } } } if(!$valid){ $form_state->setError($pane_form, $this->t('The specified coupon does not apply for this order.')); } } }
Bizim için bırakılan şey sipariş üzerine kuponu kaydetmek ve kupon işlemi yapmaktır. Bunun için, commerce_promotion_coupon işlemcisini kullanacağız.
/** * {@inheritdoc} */ public function submitPaneForm(array &$pane_form, FormStateInterface $form_state, array &$complete_form) { $values = $form_state->getValue($pane_form['#parents']); if(!empty($values['coupon'])){ $coupon_code = $values['coupon']; $commerce_coupon = $this->entityTypeManager->getStorage('commerce_promotion_coupon')->loadByCode($coupon_code); if($commerce_coupon){ $this->order->coupons[] = $commerce_coupon->id(); $coupon_order_processor = \Drupal::service('commerce_promotion.promotion_order_processor'); $coupon_order_processor->process($this->order); $this->order->save(); } } }
Bitirdik ve yeni ödeme bölmesini test edebiliriz. Mutlu kodlamalar